Svelte简单入门指南:从项目搭建到组件交互与路由配置
- 1109字
- 6分钟
- 2024-06-29
本文将详细介绍如何使用 Vite 搭建一个 Svelte + TypeScript 项目,并涵盖组件交互(父子组件、组件间的数据传递和事件传递)、Store 的多种使用方式以及路由配置等内容。
什么是 Svelte?
Svelte 是一种现代的前端框架,与传统的框架(如 React 和 Vue)不同,Svelte 在构建时将组件编译为高效的原生 JavaScript 代码,而不是在运行时解释。这样做的好处是减少了框架的开销,提升了性能,并简化了代码结构。
Svelte 可以做什么?
Svelte 可以用于构建现代的、响应式的前端应用程序。它支持组件化开发、状态管理、路由等现代前端开发所需的功能。以下是一些常见的应用场景:
- 单页面应用(SPA)
- 复杂的表单和数据处理
- 实时数据更新的仪表盘
- 交互性强的用户界面
Svelte 的优势
- 高性能:由于 Svelte 在构建时将代码编译为原生 JavaScript,减少了运行时的开销,提升了性能。
- 简洁的语法:Svelte 的语法简洁易懂,减少了样板代码,使开发更加高效。
- 无虚拟 DOM:Svelte 直接操作 DOM,而不是通过虚拟 DOM,这使得更新更加高效。
- 轻量级:生成的代码体积较小,加载速度更快。
Svelte 与 Vue、React 的对比
编译时 vs 运行时
- Svelte:在构建时将代码编译为高效的原生 JavaScript。
- React 和 Vue:在运行时解释代码,通过虚拟 DOM 进行更新。
语法和开发体验
- Svelte:语法简洁,减少了样板代码,易于上手。
- React:使用 JSX 语法,灵活但相对复杂。
- Vue:使用模板语法,介于 Svelte 和 React 之间,易于理解。
性能
- Svelte:由于编译时优化,性能通常优于 React 和 Vue。
- React 和 Vue:通过虚拟 DOM 提供较好的性能,但在极端情况下可能不如 Svelte。
初始化项目
首先,使用 Vite 创建一个新的 Svelte 项目并初始化:
1npm create vite@latest svelte-ts-app -- --template svelte-ts2cd svelte-ts-app3npm install
项目结构
项目结构如下:
1svelte-ts-app/2├── node_modules/3├── public/4│ └── favicon.ico5├── src/6│ ├── assets/7│ ├── components/8│ │ ├── ChildComponent.svelte9│ │ ├── ParentComponent.svelte10│ │ └── SiblingComponent.svelte11│ ├── routes/12│ │ ├── Home.svelte13│ │ └── About.svelte14│ ├── stores/15│ │ └── counter.ts16│ ├── App.svelte17│ ├── main.ts18│ └── index.css19├── .gitignore20├── index.html21├── package.json22├── tsconfig.json23├── vite.config.ts24└── README.md
配置 TailwindCSS(非必须)
安装 TailwindCSS:
1npm install -D tailwindcss postcss autoprefixer2npx tailwindcss init -p
在 tailwind.config.cjs
中配置 TailwindCSS:
1/** @type {import('tailwindcss').Config} */2module.exports = {3 content: ["./src/**/*.{html,js,svelte,ts}"],4 theme: {5 extend: {},6 },7 plugins: [],8};
在 src
目录下创建一个 index.css
文件,并添加以下内容:
1@tailwind base;2@tailwind components;3@tailwind utilities;
在 main.ts
中引入这个 CSS 文件:
1import "./index.css";2import App from "./App.svelte";3
4const app = new App({5 target: document.body,6});7
8export default app;
创建组件
ChildComponent.svelte
1<script lang="ts">2 import { createEventDispatcher } from "svelte";3
4 export let message: string;5 const dispatch = createEventDispatcher();6
7 function handleClick() {8 dispatch("notify", { text: "Hello from ChildComponent!" });9 }10</script>11
12<div class="p-4 bg-blue-100 rounded">13 <p>{message}</p>14 <button15 on:click={handleClick}16 class="mt-2 bg-blue-500 text-white px-4 py-2 rounded">Notify Parent</button17 >18</div>
ParentComponent.svelte
1<script lang="ts">2 import ChildComponent from "./ChildComponent.svelte";3 import SiblingComponent from "./SiblingComponent.svelte";4
5 let parentMessage = "Message from ParentComponent";6 let childMessage = "";7
8 function handleNotify(event) {9 childMessage = event.detail.text;10 }11</script>12
13<div class="p-4 bg-green-100 rounded">14 <p>{parentMessage}</p>15 <ChildComponent16 message="Message from ParentComponent to ChildComponent"17 on:notify={handleNotify}18 />19 <SiblingComponent message={childMessage} />20</div>
SiblingComponent.svelte
1<script lang="ts">2 export let message: string;3</script>4
5<div class="p-4 bg-yellow-100 rounded">6 <p>{message}</p>7</div>
使用 Store
在 Svelte 中,Store 是一种用于管理应用状态的机制,类似于 Vuex(Vue)和 Redux(React)。Svelte 提供了 writable
、readable
和 derived
等多种类型的 Store 来满足不同的需求。
stores/counter.ts
1import { writable } from "svelte/store";2
3export const counter = writable(0);
在组件中使用 Store
1<script lang="ts">2 import { counter } from "../stores/counter";3</script>4
5<div class="p-4 bg-red-100 rounded">6 <button7 on:click={() => ($counter += 1)}8 class="bg-red-500 text-white px-4 py-2 rounded">Increment</button9 >10 <p>Counter: {$counter}</p>11</div>
路由使用
安装 SvelteKit 路由
1npm install @sveltejs/kit
配置路由
在 src/routes
目录下创建路由组件:
Home.svelte
1<script lang="ts">2 import ParentComponent from "../components/ParentComponent.svelte";3</script>4
5<div class="p-4">6 <h1 class="text-2xl">Home</h1>7 <ParentComponent />8</div>
About.svelte
1<script lang="ts">2 import { counter } from "../stores/counter";3</script>4
5<div class="p-4">6 <h1 class="text-2xl">About</h1>7 <p>Counter: {$counter}</p>8</div>
配置路由
在 src/App.svelte
中配置路由:
1<script lang="ts">2 import { Router, Route } from "@sveltejs/kit";3 import Home from "./routes/Home.svelte";4 import About from "./routes/About.svelte";5</script>6
7<Router>8 <Route path="/" component={Home} />9 <Route path="/about" component={About} />10</Router>
运行项目
确保你已经安装了所有依赖,并运行项目:
1npm run dev
总结
- 组件交互:展示了父子组件之间的消息传递和事件传递,以及兄弟组件之间的数据传递。
- Store 的使用:展示了如何使用 Svelte 的 writable store 来管理全局状态。
- 路由使用:展示了如何使用 SvelteKit 路由来配置和使用路由。
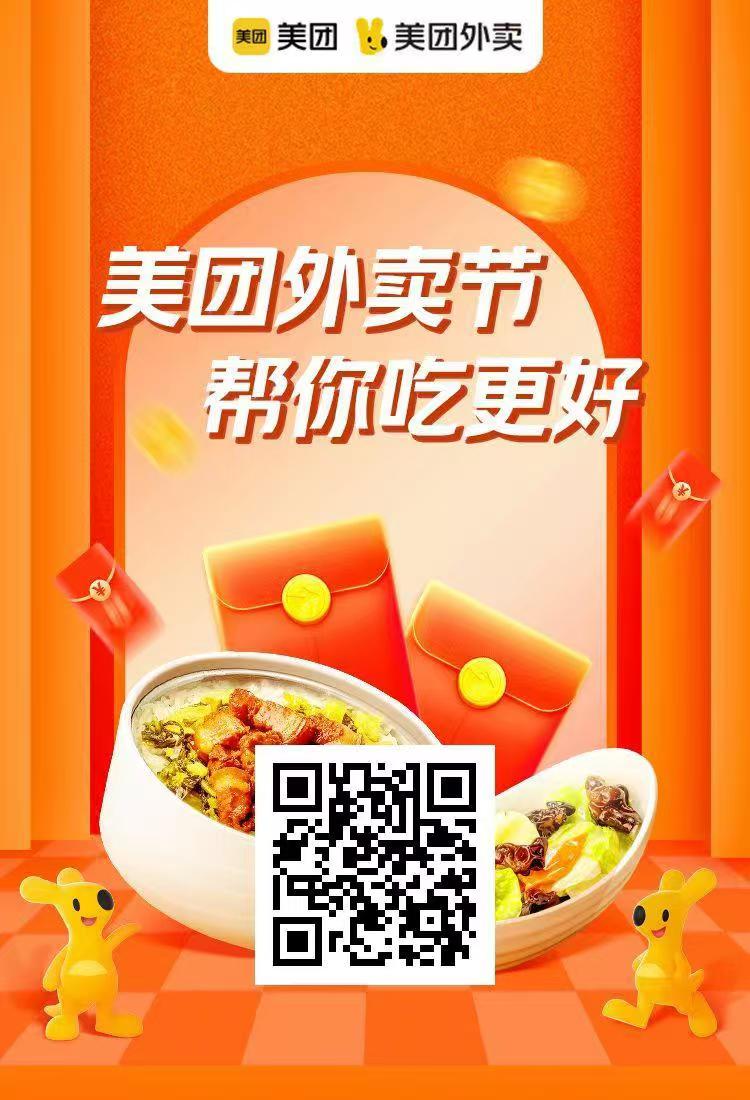
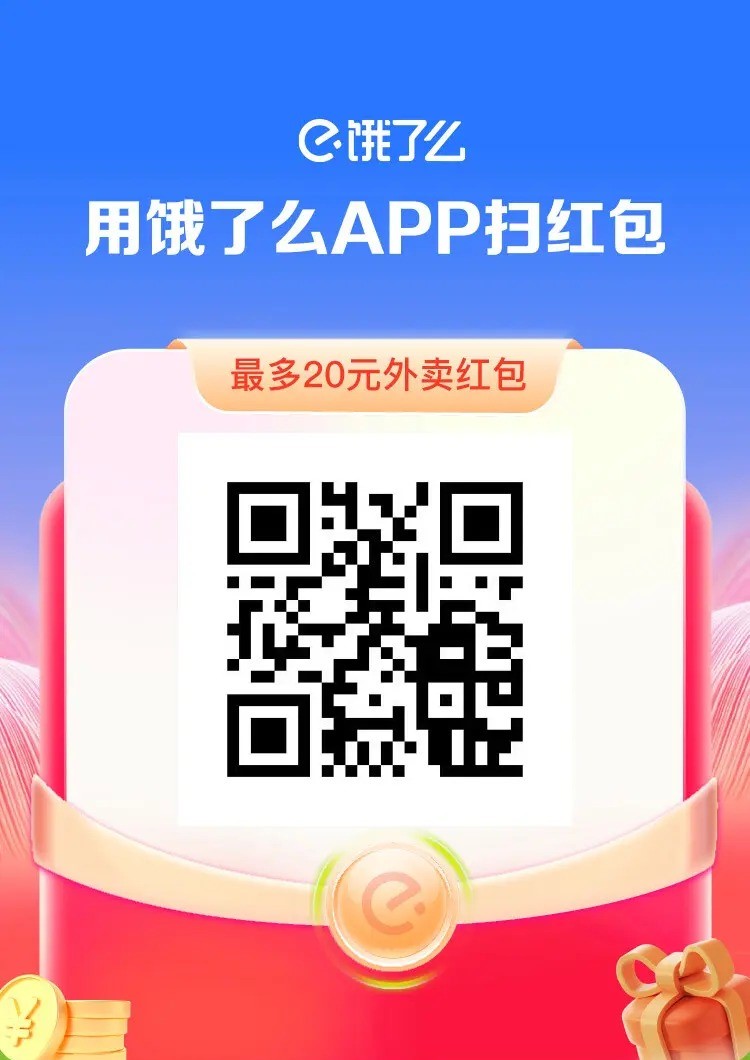
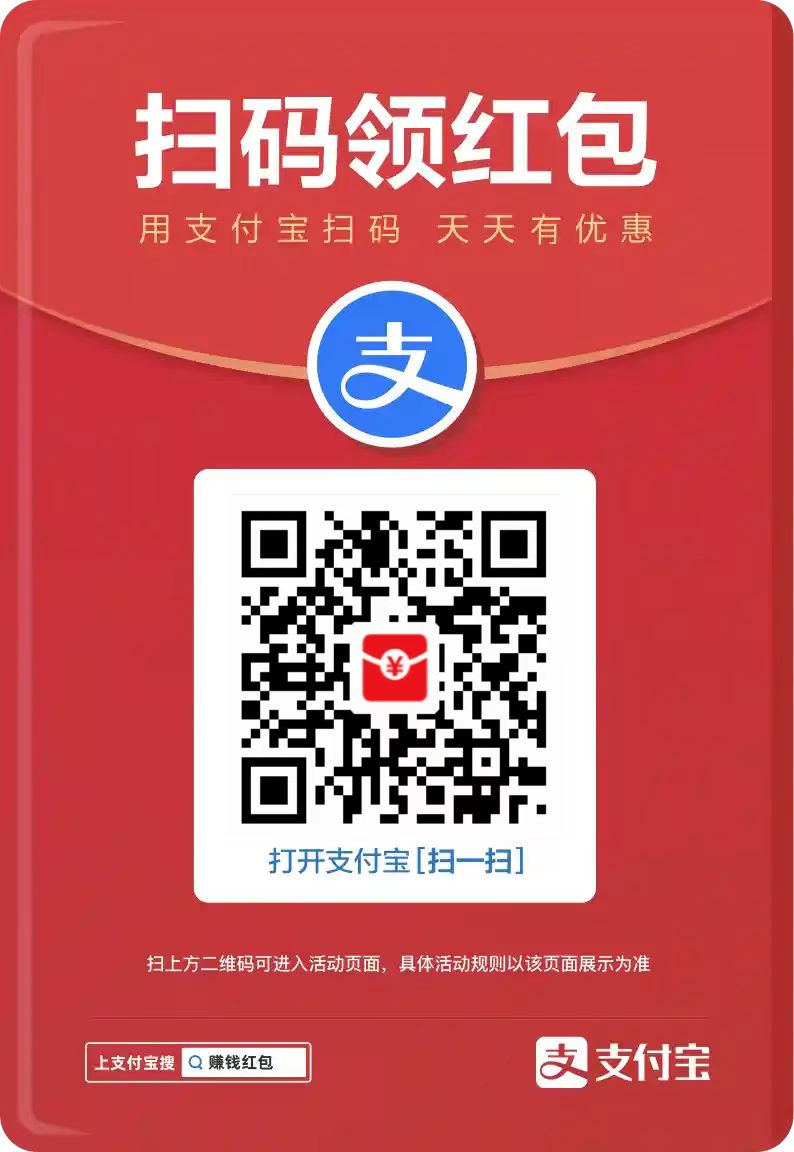