使用 Node.js 脚本自动搬运文件
- 746字
- 4分钟
- 2024-07-19
最近遇到一个需求,需要搬运指定目录下的一些文件到其它目录。具体如下:假设用户需要搬运的文件是 a.txt
,我们需要找到当前目录下存在的其它包含前缀的 a.txt
文件,例如 gz-a.txt
、dj-a.txt
、ny-a.txt
等等,然后将这些文件搬运到对应的目标目录,并重命名为 a.txt
。搬运完后,删除源目录中的原文件。
目录结构示例
源目录(用户输入的源目录路径,例如 /www/zk-prac/aa/bb/cc
)中可能包含以下文件:
1/www/zk-prac/aa/bb/cc/a.txt2/www/zk-prac/aa/bb/cc/gz-a.txt3/www/zk-prac/aa/bb/cc/dj-a.txt4/www/zk-prac/aa/bb/cc/ny-a.txt
目标基本目录(用户输入的目标基本目录路径,例如 /www/
)的结构如下:
1/www/gz-prac/aa/bb/cc/2/www/dj-prac/aa/bb/cc/3/www/ny-prac/aa/bb/cc/
在这个例子中,脚本需要将 /www/zk-prac/aa/bb/cc/gz-a.txt
搬运到 /www/gz-prac/aa/bb/cc/a.txt
,/www/zk-prac/aa/bb/cc/dj-a.txt
搬运到 /www/dj-prac/aa/bb/cc/a.txt
和/www/zk-prac/aa/bb/cc/ny-a.txt
搬运到 /www/ny-prac/aa/bb/cc/a.txt
并删除源文件。
脚本实现
安装两个依赖包:
1pnpm add inquirer fs-extra
由于使用的是ES Module,你需要在package.json
添加如下声明:
1"type": "module",
以下是实现该需求的 Node.js 脚本:
1import fs from "fs";2import path from "path";3import fse from "fs-extra";4import inquirer from "inquirer";5
6async function main() {7 // 询问用户输入源目录路径8 const { sourceDir } = await inquirer.prompt([9 {10 type: "input",11 name: "sourceDir",12 message: "请输入源目录路径:",13 validate: (input) =>14 fs.existsSync(input) ? true : "目录不存在,请输入一个有效的路径",15 },16 ]);17
18 // 询问用户输入目标基本目录路径19 const { baseTargetDir } = await inquirer.prompt([20 {21 type: "input",22 name: "baseTargetDir",23 message: "请输入目标基本目录路径:",24 validate: (input) =>25 fs.existsSync(input) ? true : "目录不存在,请输入一个有效的路径",26 },27 ]);28
29 // 询问用户输入文件名30 const { targetFileName } = await inquirer.prompt([31 {32 type: "input",33 name: "targetFileName",34 message: "请输入要搬运的文件名(例如 abc.txt):",35 validate: (input) => (input ? true : "文件名不能为空"),36 },37 ]);38
39 // 获取源目录中的所有文件40 const files = fs.readdirSync(sourceDir);41
42 // 找到所有包含前缀的目标文件43 const matchedFiles = files.filter(44 (file) => file.endsWith(targetFileName) && file !== targetFileName,45 );46
47 if (matchedFiles.length === 0) {48 console.log("未找到匹配的前缀文件。");49 return;50 }51
52 // 从源目录路径中提取相对路径部分53 const relativePath = path.relative(path.dirname(sourceDir), sourceDir);54
55 // 搬运文件到每个匹配的目标目录56 for (const file of matchedFiles) {57 const prefix = file.split("-")[0];58 const targetDirs = fs59 .readdirSync(baseTargetDir)60 .filter((dir) => dir.startsWith(prefix));61
62 for (const targetDir of targetDirs) {63 const targetPath = path.join(64 baseTargetDir,65 targetDir,66 "src",67 "content",68 "posts",69 relativePath,70 );71 const sourceFilePath = path.join(sourceDir, file);72 const targetFilePath = path.join(targetPath, targetFileName);73
74 try {75 await fse.ensureDir(targetPath);76 await fse.copy(sourceFilePath, targetFilePath);77 console.log(`已搬运 ${file} 到 ${targetFilePath}`);78 await fse.remove(sourceFilePath);79 console.log(`已删除源文件 ${sourceFilePath}`);80 } catch (err) {81 console.error(`搬运或删除文件时出错: ${err}`);82 }83 }84 }85}86
87main().catch(console.error);
使用说明
- 运行脚本时,首先会询问用户输入源目录路径。
- 然后询问用户输入目标基本目录路径。
- 用户输入要搬运的文件名(例如
a.txt
)。 - 脚本会在源目录中找到所有包含前缀的目标文件,并将它们搬运到对应的目标目录,同时将文件重命名为用户指定的文件名,并删除源文件。
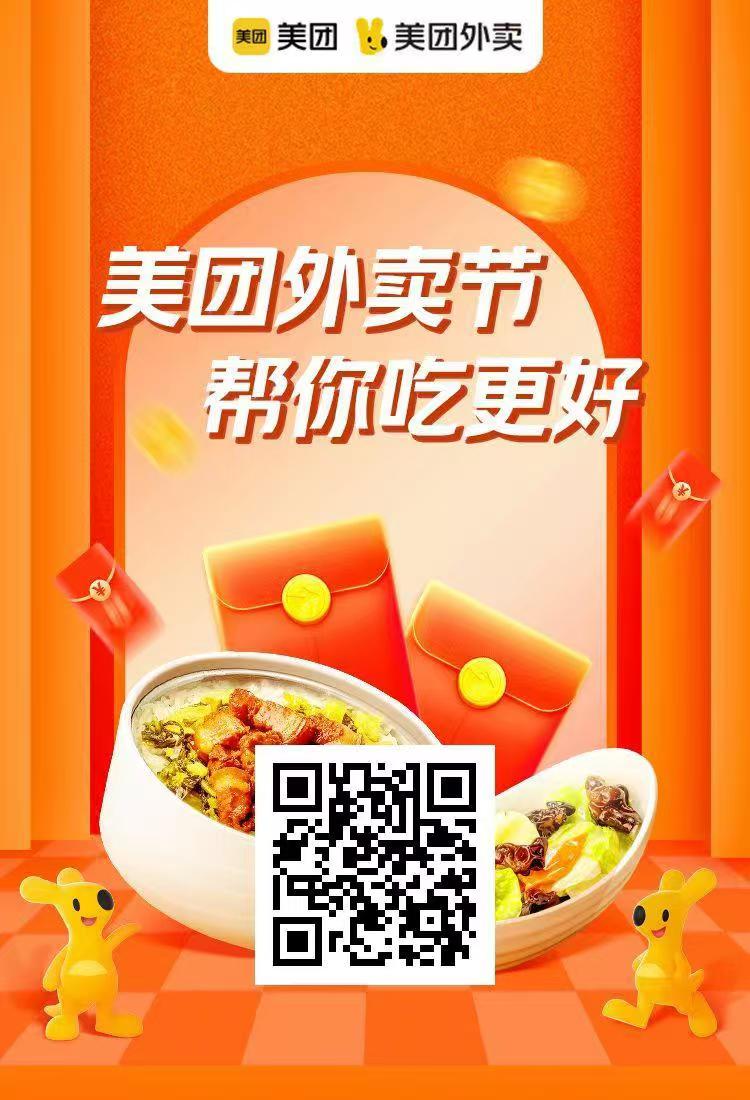
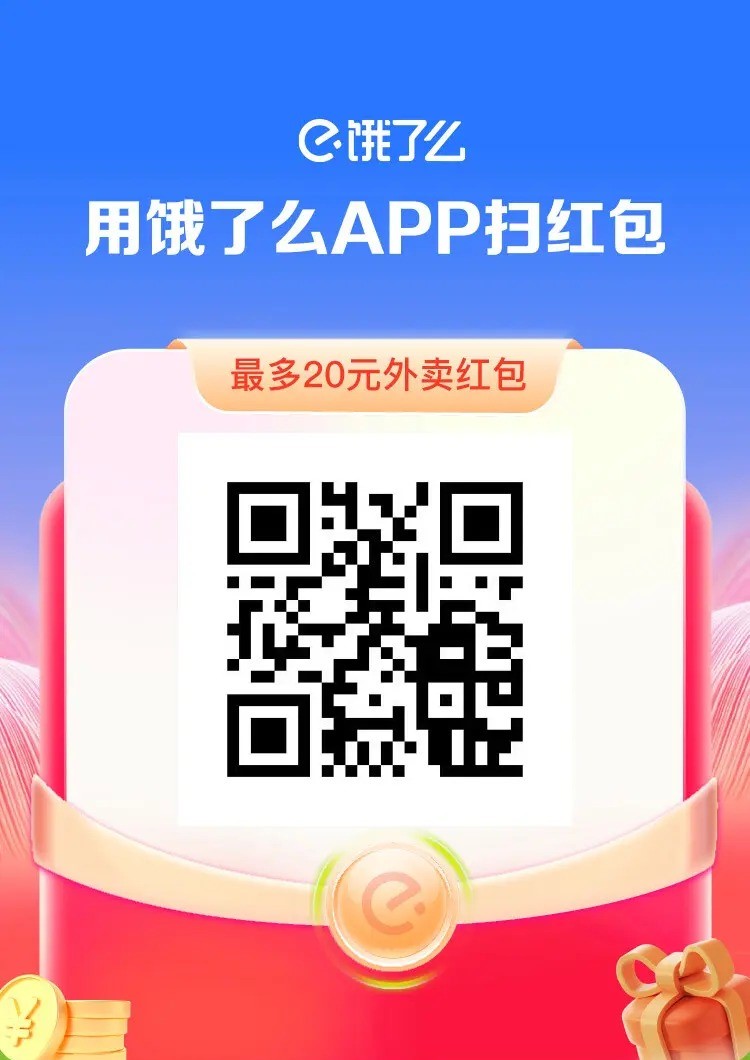
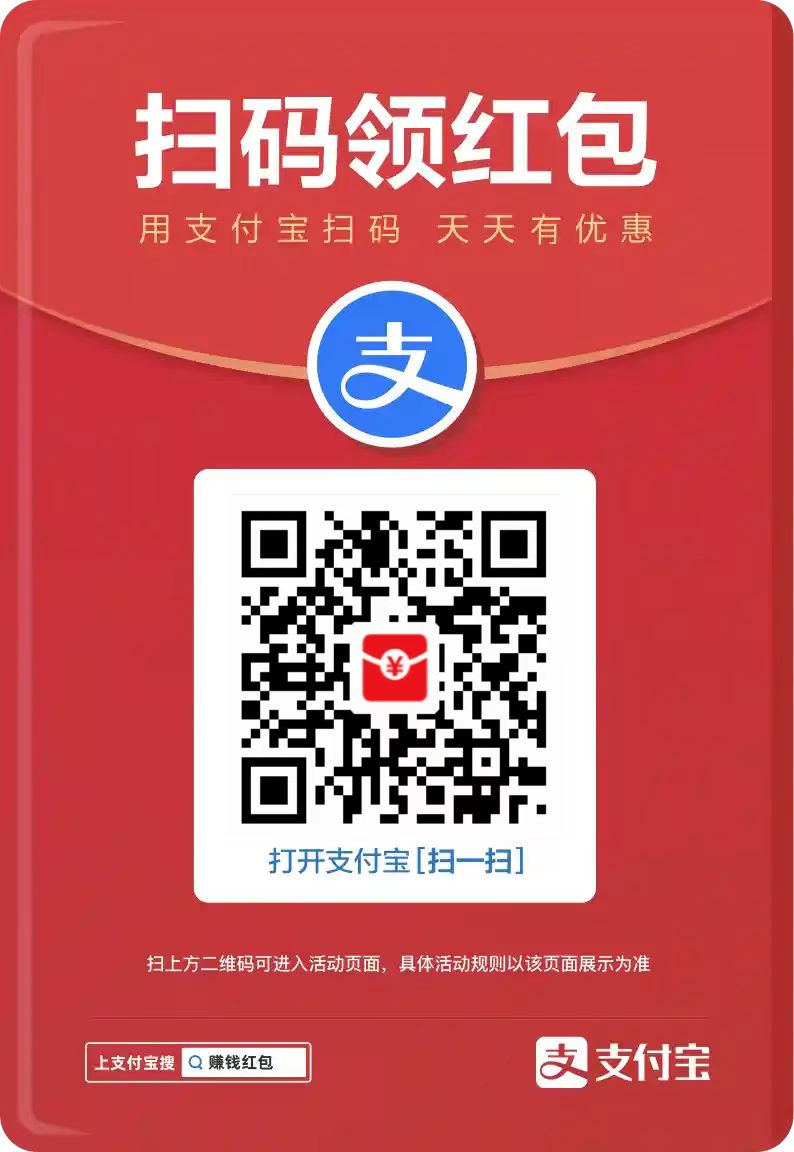