深入解析OpenAI的Function Calling:原理、应用与实践
- 1561字
- 8分钟
- 2024-06-28
OpenAI的Function Calling功能是一项强大的工具,它使得开发者能够通过自然语言调用预定义的函数。这篇文章将从what、why、how三个角度详细介绍这一功能,并结合实际应用场景给出案例代码和解释。
什么是Function Calling?
定义
Function Calling是OpenAI API的一项功能,允许开发者通过自然语言指令触发预定义的函数。通过这种方式,我们可以将复杂的操作封装在函数中,然后通过简单的自然语言调用这些函数。
工作原理
Function Calling功能依赖于OpenAI模型的自然语言理解能力。开发者定义一组函数,并将这些函数的描述传递给模型。当用户提供自然语言指令时,模型会解析这些指令并调用相应的函数。
为什么使用Function Calling?
简化复杂操作
Function Calling可以将复杂的操作封装在简单的函数调用中,使得用户无需了解底层实现细节。例如,我们可以定义一个函数来查询数据库,然后通过自然语言指令调用该函数。
提高开发效率
通过Function Calling,开发者可以更快速地构建和扩展应用程序。我们只需定义好函数和描述,剩下的工作交给OpenAI模型来完成。
增强用户体验
Function Calling使得用户可以通过自然语言与应用程序进行交互,提供更直观和友好的用户体验。这在构建聊天机器人、虚拟助手等应用中尤为有用。
如何使用Function Calling?
定义函数
首先,我们需要定义要调用的函数,并为每个函数提供描述。这些描述将帮助模型理解函数的用途和参数。
1export function getWeather(location) {2 // 假设这是一个查询天气的函数3 return `The weather in ${location} is sunny.`;4}5
6export function getNews(topic) {7 // 假设这是一个查询新闻的函数8 return `Here are the latest news about ${topic}.`;9}
配置API请求
在API请求中,我们需要传递函数描述和用户输入。模型将根据用户输入调用相应的函数。
1import { OpenAI } from "openai";2import { getWeather, getNews } from "./functions";3
4// 配置 OpenAI API5const openai = new OpenAI({6 apiKey: process.env.OPENAI_API_KEY,7});8
9// 定义函数映射10const functions = {11 getWeather,12 getNews,13};14
15// 配置模型16async function callOpenAIFunction(prompt) {17 const response = await openai.chat.completions.create({18 model: "gpt-4-0613", // 使用支持 Function Calling 的模型19 messages: [20 { role: "system", content: "You are a helpful assistant." },21 { role: "user", content: prompt },22 ],23 functions: [24 {25 name: "getWeather",26 description: "Get the current weather for a given location.",27 parameters: {28 type: "object",29 properties: {30 location: {31 type: "string",32 description: "The name of the location to get the weather for.",33 },34 },35 required: ["location"],36 },37 },38 {39 name: "getNews",40 description: "Get the latest news for a given topic.",41 parameters: {42 type: "object",43 properties: {44 topic: {45 type: "string",46 description: "The topic to get news about.",47 },48 },49 required: ["topic"],50 },51 },52 ],53 });54
55 const message = response.choices[0].message;56
57 if (message.tool_calls) {58 for (const tool_call of message.tool_calls) {59 const functionName = tool_call.function.name;60 const functionToCall = functions[functionName];61 const functionArgs = JSON.parse(tool_call.function.arguments);62 const functionResult = functionToCall(...Object.values(functionArgs));63
64 console.log(65 `Function ${functionName} called with result: ${functionResult}`,66 );67 }68 } else {69 console.log(`Model response: ${message.content}`);70 }71}72
73// 调用示例74callOpenAIFunction("Get the weather in New York.");75callOpenAIFunction("Get the latest news about technology.");
实际应用场景
场景1:智能家居控制
在智能家居系统中,我们可以定义函数来控制各个设备,并通过自然语言指令进行控制。
1export function turnOnLight(room) {2 return `Turning on the light in the ${room}.`;3}4
5export function setThermostat(temperature) {6 return `Setting thermostat to ${temperature} degrees.`;7}8
9// 配置 OpenAI API10const openai = new OpenAI({11 apiKey: process.env.OPENAI_API_KEY,12});13
14// 定义函数映射15const functions = {16 turnOnLight,17 setThermostat,18};19
20// 配置模型21async function callOpenAIFunction(prompt) {22 const response = await openai.chat.completions.create({23 model: "gpt-4-0613", // 使用支持 Function Calling 的模型24 messages: [25 { role: "system", content: "You are a helpful assistant." },26 { role: "user", content: prompt },27 ],28 functions: [29 {30 name: "turnOnLight",31 description: "Turn on the light in a specific room.",32 parameters: {33 type: "object",34 properties: {35 room: {36 type: "string",37 description: "The name of the room to turn on the light.",38 },39 },40 required: ["room"],41 },42 },43 {44 name: "setThermostat",45 description: "Set the thermostat to a specific temperature.",46 parameters: {47 type: "object",48 properties: {49 temperature: {50 type: "number",51 description: "The temperature to set the thermostat to.",52 },53 },54 required: ["temperature"],55 },56 },57 ],58 });59
60 const message = response.choices[0].message;61
62 if (message.tool_calls) {63 for (const tool_call of message.tool_calls) {64 const functionName = tool_call.function.name;65 const functionToCall = functions[functionName];66 const functionArgs = JSON.parse(tool_call.function.arguments);67 const functionResult = functionToCall(...Object.values(functionArgs));68
69 console.log(70 `Function ${functionName} called with result: ${functionResult}`,71 );72 }73 } else {74 console.log(`Model response: ${message.content}`);75 }76}77
78// 调用示例79callOpenAIFunction("Turn on the light in the living room.");80callOpenAIFunction("Set the thermostat to 22 degrees.");
场景2:客户支持
在客户支持系统中,我们可以定义函数来处理常见问题,并通过自然语言指令进行调用。
1export function checkOrderStatus(orderId) {2 return `Order ${orderId} is currently being processed.`;3}4
5export function cancelOrder(orderId) {6 return `Order ${orderId} has been cancelled.`;7}8
9// 配置 OpenAI API10const openai = new OpenAI({11 apiKey: process.env.OPENAI_API_KEY,12});13
14// 定义函数映射15const functions = {16 checkOrderStatus,17 cancelOrder,18};19
20// 配置模型21async function callOpenAIFunction(prompt) {22 const response = await openai.chat.completions.create({23 model: "gpt-4-0613", // 使用支持 Function Calling 的模型24 messages: [25 { role: "system", content: "You are a helpful assistant." },26 { role: "user", content: prompt },27 ],28 functions: [29 {30 name: "checkOrderStatus",31 description: "Check the status of an order.",32 parameters: {33 type: "object",34 properties: {35 orderId: {36 type: "string",37 description: "The ID of the order to check.",38 },39 },40 required: ["orderId"],41 },42 },43 {44 name: "cancelOrder",45 description: "Cancel an order.",46 parameters: {47 type: "object",48 properties: {49 orderId: {50 type: "string",51 description: "The ID of the order to cancel.",52 },53 },54 required: ["orderId"],55 },56 },57 ],58 });59
60 const message = response.choices[0].message;61
62 if (message.tool_calls) {63 for (const tool_call of message.tool_calls) {64 const functionName = tool_call.function.name;65 const functionToCall = functions[functionName];66 const functionArgs = JSON.parse(tool_call.function.arguments);67 const functionResult = functionToCall(...Object.values(functionArgs));68
69 console.log(70 `Function ${functionName} called with result: ${functionResult}`,71 );72 }73 } else {74 console.log(`Model response: ${message.content}`);75 }76}77
78// 调用示例79callOpenAIFunction("Check the status of order 12345.");80callOpenAIFunction("Cancel order 12345.");
最佳实践
- 清晰的函数描述:确保每个函数的描述清晰明了,让模型能够准确理解函数的用途和参数。
- 适当的错误处理:在实际应用中,确保对可能的错误情况进行处理,如函数调用失败或参数不正确。
- 持续优化:根据用户反馈和使用情况,不断优化函数和描述,提高模型的准确性和用户体验。
结论
OpenAI的Function Calling功能通过将自然语言指令与预定义函数结合,简化了复杂操作,提高了开发效率,并增强了用户体验。无论是在智能家居、客户支持还是其他应用场景中,Function Calling都能提供强大的支持。
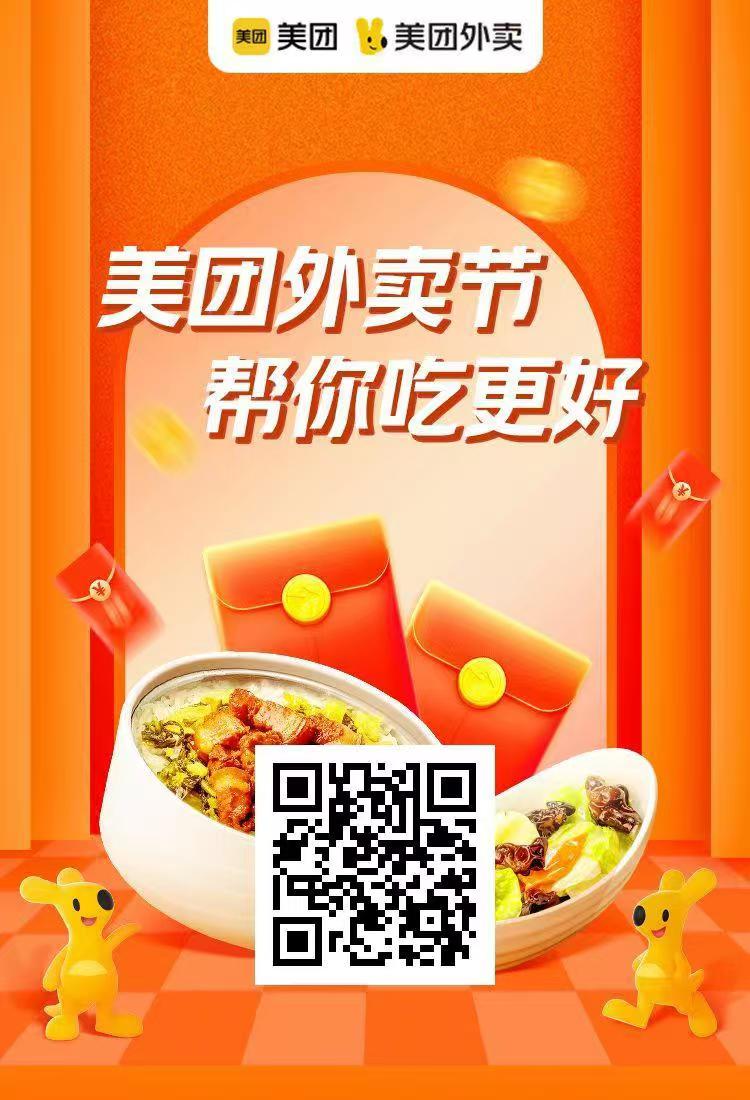
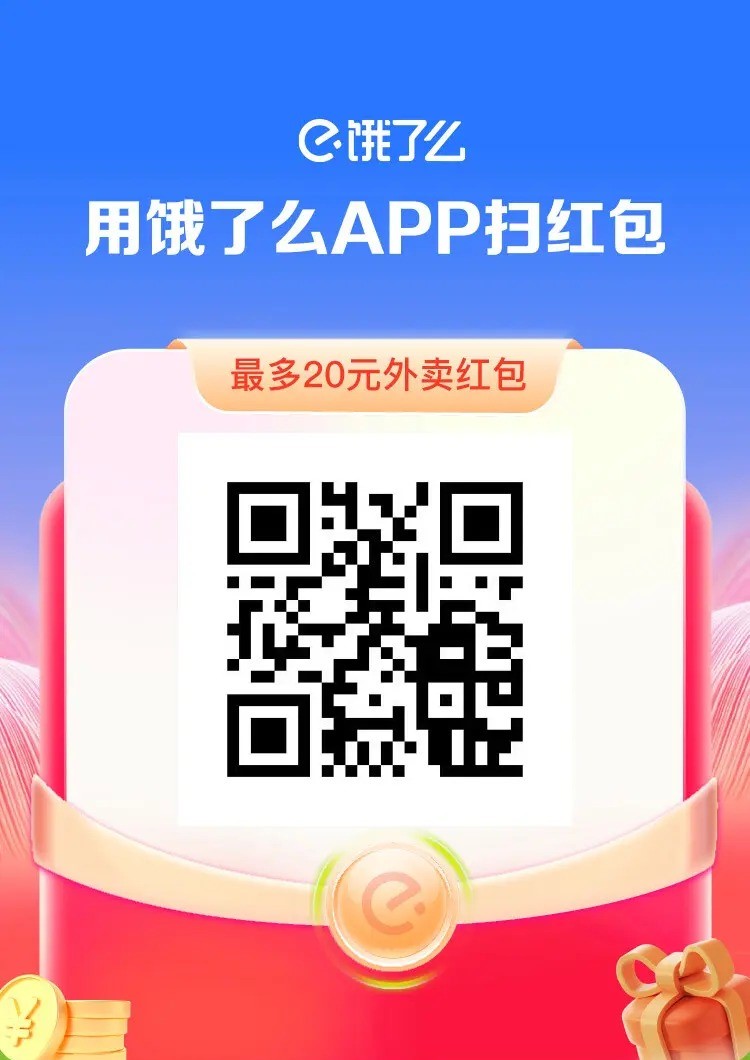
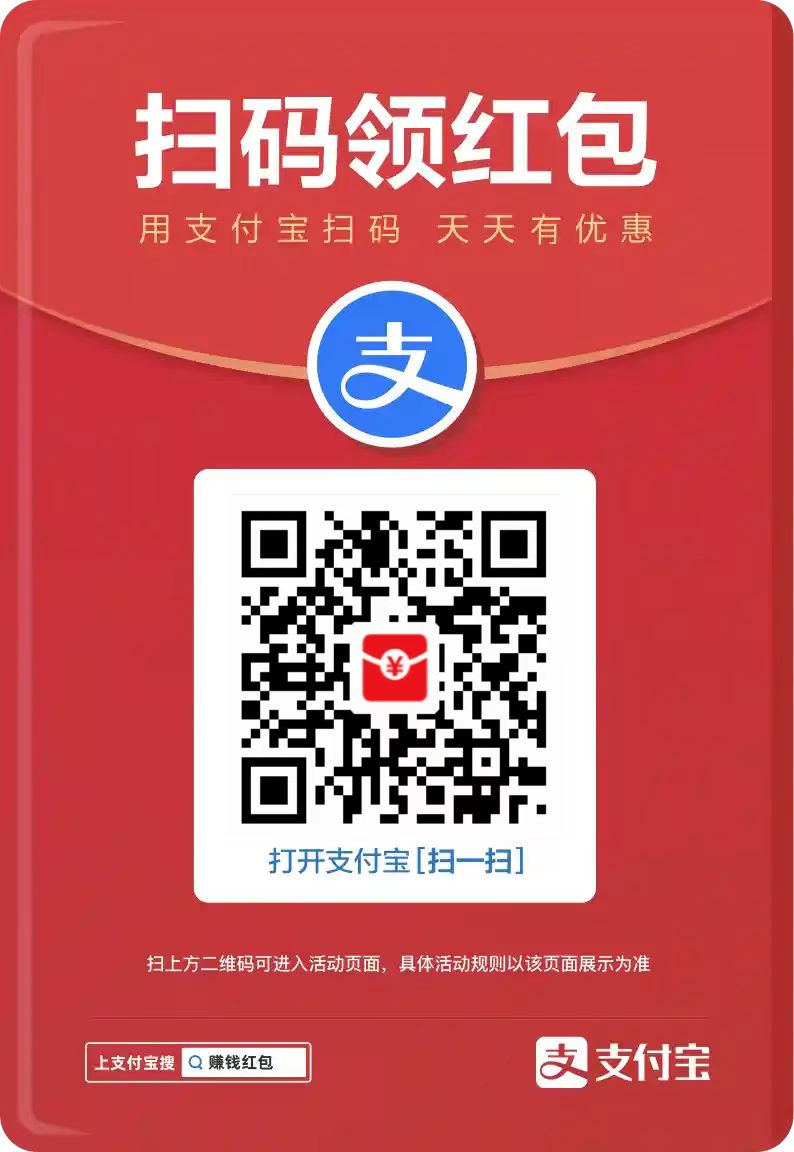